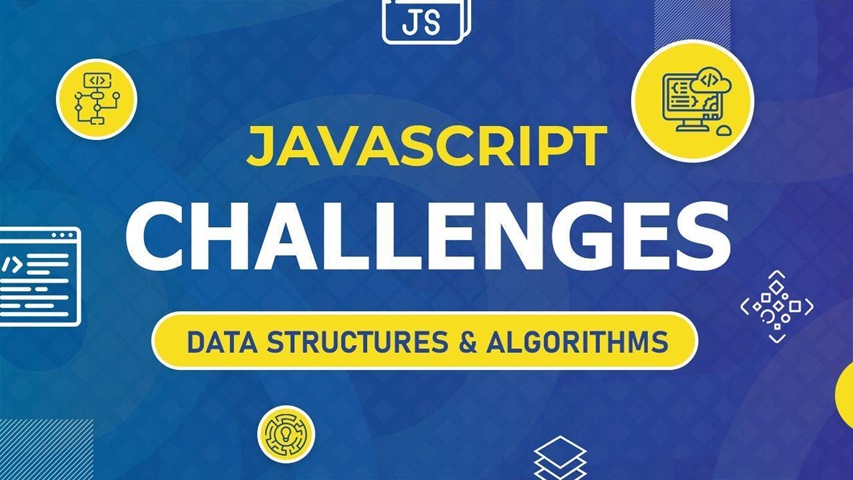
Essential JavaScript Algorithms & Data Structures: Ultimate Developer Guide
Essential JavaScript Algorithms and Data Structures: The Ultimate Developer’s Guide
Table of Contents
- Introduction
- Understanding JavaScript Data Structures
- Essential Algorithms Every JavaScript Developer Should Know
- Practical Applications in Real-World Projects
- Implementing Efficient Data Structures in JavaScript
- Common Algorithm Challenges and Solutions
- Modern JavaScript Algorithm Techniques
- Resources and Next Steps
- Conclusion
Introduction
In the ever-evolving world of web development, mastering JavaScript algorithms and data structures isn’t just for acing technical interviews—it’s the foundation of writing efficient, scalable code. Whether you’re building a responsive web application, processing large datasets, or optimizing your code for performance, understanding how to structure and manipulate data effectively is crucial.
This comprehensive guide will walk you through the essential JavaScript data structures and algorithms that every developer should know. We’ll explore practical implementations, real-world applications, and modern approaches to solving common programming challenges.
By the end of this guide, you’ll have a solid understanding of how to leverage JavaScript’s capabilities to solve complex problems efficiently. Let’s dive in and unlock the full potential of your JavaScript code!
Understanding JavaScript Data Structures
Data structures are specialized formats for organizing and storing data. In JavaScript, understanding the right data structure for your specific problem can dramatically improve your application’s performance and code readability.
Arrays: The Foundation of Collections
Arrays are the workhorses of JavaScript data structures—versatile, built-in, and powerful. They store ordered collections of items that can be accessed by index.
// Basic array operations
const technologies = ['JavaScript', 'React', 'Node.js', 'MongoDB'];
// Adding elements
technologies.push('TypeScript'); // Add to end
technologies.unshift('HTML'); // Add to beginning
// Removing elements
const lastItem = technologies.pop(); // Remove from end
const firstItem = technologies.shift(); // Remove from beginning
// Finding elements
const hasReact = technologies.includes('React'); // true
const reactIndex = technologies.indexOf('React'); // 1
Arrays shine when you need ordered data with fast access by position. However, they can become inefficient when inserting or deleting elements in the middle, as these operations require shifting elements.
Pro Tip: Use array methods like map()
, filter()
, and reduce()
for clean, functional operations on your data.
Objects: JavaScript’s Dynamic Key-Value Stores
Objects are JavaScript’s native implementation of key-value pairs, perfect for when you need to access data by a specific key rather than position.
// Creating and using objects
const developer = {
name: 'Alex',
skills: ['JavaScript', 'React', 'Node.js'],
yearsExperience: 5,
active: true
};
// Accessing properties
console.log(developer.name); // "Alex"
console.log(developer['skills']); // ["JavaScript", "React", "Node.js"]
// Adding new properties
developer.location = 'Remote';
// Checking if a property exists
const hasSkills = 'skills' in developer; // true
Objects provide constant-time access to values when you know the key, making them excellent for lookups. However, they don’t maintain insertion order (prior to ES2015) and aren’t ideal for ordered data.
Maps and Sets: Modern Collection Types
ES6 introduced Maps and Sets, providing more powerful alternatives to objects and arrays for specific use cases.
Maps allow any data type as keys (not just strings) and maintain insertion order:
// Using Map for complex key types
const userPreferences = new Map();
// Using objects as keys
const user1 = { id: 1, name: 'Jamie' };
const user2 = { id: 2, name: 'Taylor' };
userPreferences.set(user1, { theme: 'dark', notifications: true });
userPreferences.set(user2, { theme: 'light', notifications: false });
// Retrieving values
console.log(userPreferences.get(user1)); // { theme: 'dark', notifications: true }
Sets store unique values and provide efficient membership testing:
// Using Set for unique collections
const visitedPages = new Set();
// Adding values
visitedPages.add('/home');
visitedPages.add('/products');
visitedPages.add('/home'); // Duplicate - will not be added
// Checking membership
if (visitedPages.has('/home')) {
console.log('Already visited the home page');
}
// Size of unique pages
console.log(visitedPages.size); // 2
Maps and Sets offer better performance for specific operations compared to objects and arrays. Use Maps when you need key-value pairs with non-string keys or need to maintain insertion order, and Sets when you need to store unique values.
Stacks and Queues: Managing Ordered Data
While not built-in, stacks and queues are essential abstract data structures easily implemented in JavaScript.
Stacks follow the Last-In-First-Out (LIFO) principle:
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
if (this.isEmpty()) return "Underflow";
return this.items.pop();
}
peek() {
return this.items[this.items.length - 1];
}
isEmpty() {
return this.items.length === 0;
}
}
// Using our stack
const browserHistory = new Stack();
browserHistory.push('/home');
browserHistory.push('/products');
browserHistory.push('/product/123');
// Go back
const previousPage = browserHistory.pop(); // '/product/123'
Stacks are ideal for tracking history, managing function calls, and parsing expressions.
Queues follow the First-In-First-Out (FIFO) principle:
class Queue {
constructor() {
this.items = [];
}
enqueue(element) {
this.items.push(element);
}
dequeue() {
if (this.isEmpty()) return "Underflow";
return this.items.shift();
}
front() {
if (this.isEmpty()) return "Queue is empty";
return this.items[0];
}
isEmpty() {
return this.items.length === 0;
}
}
// Using our queue
const taskQueue = new Queue();
taskQueue.enqueue('Send email notification');
taskQueue.enqueue('Process payment');
taskQueue.enqueue('Update inventory');
// Process next task
const nextTask = taskQueue.dequeue(); // 'Send email notification'
Queues excel in scheduling, resource management, and handling asynchronous operations.
Linked Lists: Dynamic Memory Allocation
Linked lists consist of nodes, each containing data and a reference to the next node. They provide dynamic memory allocation and efficient insertions/deletions.
class Node {
constructor(data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.size = 0;
}
append(data) {
const newNode = new Node(data);
if (!this.head) {
this.head = newNode;
} else {
let current = this.head;
while (current.next) {
current = current.next;
}
current.next = newNode;
}
this.size++;
}
insertAt(data, index) {
if (index < 0 || index > this.size) {
return false;
}
const newNode = new Node(data);
if (index === 0) {
newNode.next = this.head;
this.head = newNode;
} else {
let current = this.head;
let previous = null;
let count = 0;
while (count < index) {
previous = current;
current = current.next;
count++;
}
newNode.next = current;
previous.next = newNode;
}
this.size++;
return true;
}
}
// Using our linked list
const playlist = new LinkedList();
playlist.append("Bohemian Rhapsody");
playlist.append("Stairway to Heaven");
playlist.insertAt("Sweet Child O' Mine", 1);
Linked lists excel when you need constant-time insertions/deletions from the list and don’t need random access to elements.
Trees and Graphs: Hierarchical Relationships
Trees and graphs represent hierarchical and network relationships respectively.
Binary Search Trees (BST) organize data for efficient searching:
class TreeNode {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
class BinarySearchTree {
constructor() {
this.root = null;
}
insert(value) {
const newNode = new TreeNode(value);
if (!this.root) {
this.root = newNode;
return this;
}
let current = this.root;
while (true) {
if (value === current.value) return undefined;
if (value < current.value) {
if (!current.left) {
current.left = newNode;
return this;
}
current = current.left;
} else {
if (!current.right) {
current.right = newNode;
return this;
}
current = current.right;
}
}
}
find(value) {
if (!this.root) return false;
let current = this.root;
let found = false;
while (current && !found) {
if (value < current.value) {
current = current.left;
} else if (value > current.value) {
current = current.right;
} else {
found = true;
}
}
if (!found) return false;
return current;
}
}
// Using our BST
const searchTree = new BinarySearchTree();
searchTree.insert(10);
searchTree.insert(5);
searchTree.insert(15);
searchTree.insert(2);
searchTree.insert(7);
const node = searchTree.find(7); // Returns the node with value 7
Trees are excellent for representing hierarchical data like file systems, organization charts, and for efficient searching.
Essential Algorithms Every JavaScript Developer Should Know
Now that we’ve covered data structures, let’s explore the algorithms that help us manipulate and process data efficiently.
Sorting Algorithms in JavaScript
Sorting is one of the most common operations in programming. Here are some key sorting algorithms:
Bubble Sort: Simple but inefficient for large datasets.
function bubbleSort(arr) {
const n = arr.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap elements
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
const numbers = [64, 34, 25, 12, 22, 11, 90];
bubbleSort(numbers); // [11, 12, 22, 25, 34, 64, 90]
Quick Sort: Much more efficient, using a divide-and-conquer approach.
function quickSort(arr) {
if (arr.length <= 1) {
return arr;
}
const pivot = arr[arr.length - 1];
const left = [];
const right = [];
for (let i = 0; i < arr.length - 1; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
const numbers = [64, 34, 25, 12, 22, 11, 90];
quickSort(numbers); // [11, 12, 22, 25, 34, 64, 90]
JavaScript’s built-in Array.prototype.sort()
method is typically implemented using a variant of quick sort or merge sort, depending on the browser.
Searching Algorithms: Finding What You Need
Efficient search algorithms are crucial for finding data in collections:
Linear Search: Checks each element sequentially.
function linearSearch(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) {
return i;
}
}
return -1;
}
const numbers = [64, 34, 25, 12, 22, 11, 90];
linearSearch(numbers, 22); // 4
Binary Search: Much faster for sorted arrays, using a divide-and-conquer approach.
function binarySearch(sortedArr, target) {
let left = 0;
let right = sortedArr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (sortedArr[mid] === target) {
return mid;
}
if (sortedArr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
const sortedNumbers = [11, 12, 22, 25, 34, 64, 90];
binarySearch(sortedNumbers, 22); // 2
Binary search is extremely efficient with O(log n) time complexity, but requires the array to be sorted first.
Dynamic Programming: Solving Complex Problems
Dynamic programming breaks down complex problems into simpler subproblems, solving each subproblem once and storing the solution.
A classic example is calculating Fibonacci numbers:
// Without dynamic programming (inefficient)
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// With dynamic programming (memoization)
function fibonacciDP(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacciDP(n - 1, memo) + fibonacciDP(n - 2, memo);
return memo[n];
}
// Even more efficient (bottom-up)
function fibonacciBottomUp(n) {
if (n <= 1) return n;
let fib = [0, 1];
for (let i = 2; i <= n; i++) {
fib[i] = fib[i - 1] + fib[i - 2];
}
return fib[n];
}
console.log(fibonacciDP(40)); // Quick calculation
console.log(fibonacci(40)); // Much slower
Dynamic programming is essential for optimization problems, like finding the shortest path or maximizing value within constraints.
Recursion: Elegant Problem-Solving
Recursion is a technique where a function calls itself to solve a problem. It’s particularly useful for tasks that can be broken down into similar subtasks.
// Calculating factorial using recursion
function factorial(n) {
// Base case
if (n === 0 || n === 1) {
return 1;
}
// Recursive case
return n * factorial(n - 1);
}
console.log(factorial(5)); // 120 (5 * 4 * 3 * 2 * 1)
Recursion provides elegant solutions for tree traversal, nested data processing, and divide-and-conquer algorithms. However, be mindful of the call stack size limitations in JavaScript.
Practical Applications in Real-World Projects
Let’s explore how these algorithms and data structures apply to everyday development challenges.
Web Application Performance Optimization
Efficient algorithms dramatically improve user experience:
- Virtual List Implementation: Using windowing techniques to render only visible items in long lists:
function VirtualList({ items, itemHeight, windowHeight }) {
const [scrollTop, setScrollTop] = useState(0);
// Calculate visible range
const startIndex = Math.floor(scrollTop / itemHeight);
const endIndex = Math.min(
items.length - 1,
Math.floor((scrollTop + windowHeight) / itemHeight)
);
// Only render visible items
const visibleItems = items.slice(startIndex, endIndex + 1).map((item, index) => (
<div
key={startIndex + index}
style={{
position: 'absolute',
top: (startIndex + index) * itemHeight,
height: itemHeight
}}
>
{item.content}
</div>
));
return (
<div
style={{ height: windowHeight, overflow: 'auto' }}
onScroll={(e) => setScrollTop(e.currentTarget.scrollTop)}
>
<div style={{ height: items.length * itemHeight, position: 'relative' }}>
{visibleItems}
</div>
</div>
);
}
- Memoization for Expensive Calculations: Caching results of expensive operations:
// Using memoization to optimize expensive calculations
function memoize(fn) {
const cache = new Map();
return function(...args) {
const key = JSON.stringify(args);
if (cache.has(key)) {
return cache.get(key);
}
const result = fn.apply(this, args);
cache.set(key, result);
return result;
};
}
// Example usage
const expensiveCalculation = memoize((a, b) => {
console.log('Calculating...');
// Simulate expensive operation
let result = 0;
for (let i = 0; i < 1000000; i++) {
result += a * b;
}
return result;
});
console.log(expensiveCalculation(4, 5)); // Calculates
console.log(expensiveCalculation(4, 5)); // Returns cached result
Data Processing and Manipulation
Efficient algorithms make data transformation and analysis more manageable:
- Map-Reduce for Data Transformation: Processing large datasets:
// Sample sales data
const sales = [
{ product: 'Laptop', amount: 1200, category: 'Electronics' },
{ product: 'Headphones', amount: 100, category: 'Electronics' },
{ product: 'Book', amount: 15, category: 'Books' },
{ product: 'Smartphone', amount: 800, category: 'Electronics' },
{ product: 'Coffee Mug', amount: 10, category: 'Kitchen' }
];
// Map: Transform data
const mappedSales = sales.map(sale => ({
...sale,
tax: sale.amount * 0.08,
total: sale.amount * 1.08
}));
// Reduce: Calculate totals by category
const salesByCategory = sales.reduce((acc, sale) => {
// If category doesn't exist in accumulator, initialize it
if (!acc[sale.category]) {
acc[sale.category] = 0;
}
// Add sale amount to category total
acc[sale.category] += sale.amount;
return acc;
}, {});
console.log(salesByCategory);
// { Electronics: 2100, Books: 15, Kitchen: 10 }
Front-End UI Rendering Techniques
Modern JS frameworks use sophisticated algorithms for efficient DOM updates:
- Diffing Algorithms: Similar to those used in React’s Virtual DOM:
// Simplified version of a diffing algorithm
function updateElement(parent, newNode, oldNode, index = 0) {
// If old node doesn't exist, simply append the new node
if (!oldNode) {
parent.appendChild(createElement(newNode));
}
// If new node doesn't exist, remove the old node
else if (!newNode) {
parent.removeChild(parent.childNodes[index]);
}
// If nodes are different, replace old with new
else if (changed(newNode, oldNode)) {
parent.replaceChild(createElement(newNode), parent.childNodes[index]);
}
// If nodes are the same type, update children
else if (newNode.type) {
const newLength = newNode.children.length;
const oldLength = oldNode.children.length;
// Update all children that exist in both new and old
for (let i = 0; i < newLength || i < oldLength; i++) {
updateElement(
parent.childNodes[index],
newNode.children[i],
oldNode.children[i],
i
);
}
}
}
Implementing Efficient Data Structures in JavaScript
Building Custom Data Structures
Creating specialized data structures can significantly improve performance for specific use cases:
- Trie for Autocomplete: Efficient prefix searching:
class TrieNode {
constructor() {
this.children = {};
this.isEndOfWord = false;
}
}
class Trie {
constructor() {
this.root = new TrieNode();
}
insert(word) {
let current = this.root;
for (const char of word) {
if (!current.children[char]) {
current.children[char] = new TrieNode();
}
current = current.children[char];
}
current.isEndOfWord = true;
}
search(word) {
let current = this.root;
for (const char of word) {
if (!current.children[char]) {
return false;
}
current = current.children[char];
}
return current.isEndOfWord;
}
startsWith(prefix) {
let current = this.root;
for (const char of prefix) {
if (!current.children[char]) {
return false;
}
current = current.children[char];
}
return true;
}
findWordsWithPrefix(prefix) {
const result = [];
let current = this.root;
// Navigate to the end of the prefix
for (const char of prefix) {
if (!current.children[char]) {
return result;
}
current = current.children[char];
}
// Helper function to find all words from current node
function findWords(node, currentWord) {
if (node.isEndOfWord) {
result.push(currentWord);
}
for (const char in node.children) {
findWords(node.children[char], currentWord + char);
}
}
findWords(current, prefix);
return result;
}
}
// Using our Trie for autocomplete
const searchTrie = new Trie();
const words = ['apple', 'application', 'apply', 'banana', 'ball', 'cat'];
words.forEach(word => searchTrie.insert(word));
console.log(searchTrie.findWordsWithPrefix('app')); // ['apple', 'application', 'apply']
console.log(searchTrie.findWordsWithPrefix('ba')); // ['banana', 'ball']
Memory Management Considerations
JavaScript’s automatic memory management doesn’t absolve developers from considering memory usage:
- Avoid Memory Leaks: Be careful with closures and event listeners:
// Potential memory leak
function createButtons() {
const data = new Array(10000).fill('Some large data');
document.getElementById('button').addEventListener('click', function() {
// This closure captures the entire 'data' array
console.log('Button clicked, data length:', data.length);
});
}
// Better approach
function createButtonsEfficient() {
const data = new Array(10000).fill('Some large data');
const dataSize = data.length; // Extract only what's needed
document.getElementById('button').addEventListener('click', function() {
// This closure only captures the size, not the entire array
console.log('Button clicked, data length:', dataSize);
});
// The data array can now be garbage collected if no longer needed
}
Common Algorithm Challenges and Solutions
Time and Space Complexity Tradeoffs
Understanding Big O notation helps make informed decisions about algorithm selection:
Algorithm | Time Complexity (Average) | Space Complexity | Best Use Case |
---|---|---|---|
Bubble Sort | O(n²) | O(1) | Small datasets, nearly sorted data |
Quick Sort | O(n log n) | O(log n) | General purpose sorting |
Binary Search | O(log n) | O(1) | Finding items in sorted arrays |
Hash Table Lookup | O(1) | O(n) | Fast lookups when key is known |
Example of space-time tradeoff:
// Time-efficient but space-intensive approach (memoization)
function nthFibonacci(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = nthFibonacci(n - 1, memo) + nthFibonacci(n - 2, memo);
return memo[n];
}
// Space-efficient but time-intensive approach
function nthFibonacciIterative(n) {
if (n <= 1) return n;
let a = 0, b = 1;
for (let i = 2; i <= n; i++) {
const temp = a + b;
a = b;
b = temp;
}
return b;
}
Debugging Algorithm Implementations
Tips for troubleshooting algorithm code:
- Use small, manageable test cases first
- Track variable states with console.log at critical points
- Visualize algorithm execution with tools like visualgo.net
- Use time complexity analysis to identify bottlenecks
function debugBubbleSort(arr) {
console.log('Initial array:', arr);
const n = arr.length;
for (let i = 0; i < n; i++) {
console.log(`Outer loop iteration ${i + 1}:`);
let swapped = false;
for (let j = 0; j < n - i - 1; j++) {
console.log(` Comparing ${arr[j]} and ${arr[j + 1]}`);
if (arr[j] > arr[j + 1]) {
// Swap elements
console.log(` Swapping ${arr[j]} and ${arr[j + 1]}`);
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
swapped = true;
console.log(` Array now: ${arr}`);
}
}
if (!swapped) {
console.log(' No swaps needed, array is sorted');
break;
}
}
console.log('Final sorted array:', arr);
return arr;
}
Modern JavaScript Algorithm Techniques
Using ES6+ Features for Cleaner Algorithms
Modern JavaScript features make algorithm implementation more concise and readable:
// Using destructuring for swapping values
function quickSortES6(arr) {
if (arr.length <= 1) return arr;
const pivot = arr[0];
const [, ...rest] = arr;
const left = rest.filter(x => x < pivot);
const right = rest.filter(x => x >= pivot);
return [...quickSortES6(left), pivot, ...quickSortES6(right)];
}
// Using map and reduce for cleaner data processing
function analyzeScores(students) {
const scores = students.map(student => student.score);
const stats = {
average: scores.reduce((sum, score) => sum + score, 0) / scores.length,
highest: Math.max(...scores),
lowest: Math.min(...scores),
passing: scores.filter(score => score >= 60).length
};
return stats;
}
Functional Programming Approaches
Functional programming paradigms can lead to more maintainable, testable algorithm implementations:
// Pure function for calculating factorial
const factorial = n => n <= 1 ? 1 : n * factorial(n - 1);
// Compose higher-order functions
const pipe = (...fns) => x => fns.reduce((y, f) => f(y), x);
// Create a data processing pipeline
const processData = pipe(
data => data.filter(item => item.active),
data => data.map(item => ({ ...item, score: item.raw * 10 })),
data => data.sort((a, b) => b.score - a.score),
data => data.slice(0, 5) // Top 5 results
);
// Apply the pipeline to our data
const topResults = processData(rawData);
Resources and Next Steps
To continue mastering JavaScript algorithms and data structures:
Practice Platforms:
- LeetCode
- HackerRank
- CodeSignal
Advanced Topics to Explore:
- Graph algorithms (Dijkstra’s, A*)
- Advanced tree structures (AVL trees, Red-Black trees)
- Machine learning algorithms in JavaScript
Recommended Books:
- “Grokking Algorithms” by Aditya Bhargava
- “JavaScript Data Structures and Algorithms” by Sammie Bae
Conclusion
Mastering JavaScript algorithms and data structures is an ongoing journey that will significantly improve your capabilities as a developer. By understanding when and how to apply these concepts, you’ll write more efficient code, solve complex problems more elegantly, and ultimately build better applications.
Remember that the best data structure or algorithm depends entirely on your specific use case. Always consider the tradeoffs between time complexity, space complexity, and code readability when making your decisions.
Start with the fundamentals covered in this guide, practice regularly with real-world problems, and gradually work your way toward more advanced concepts. Your future self (and your users) will thank you for the performance improvements and cleaner code that result from this investment in your skills.
Comments