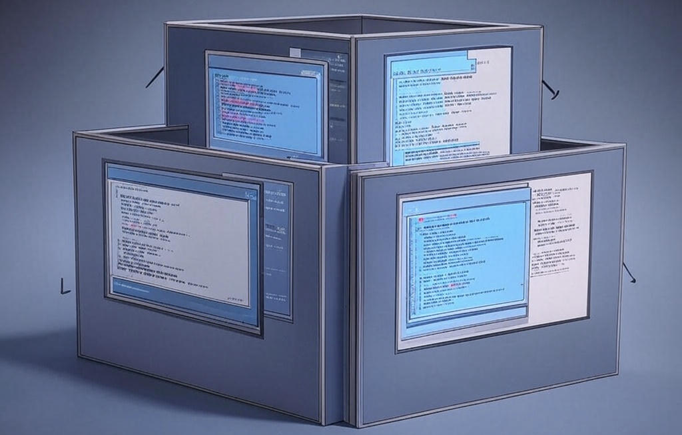
The Ultimate Guide for Modern Web Developers [2025]
JavaScript Closures: The Ultimate Guide with Practical Examples and Real-World Applications
Table of Contents
- Introduction
- What is a Closure in JavaScript?
- How Closures Work: Scope and Lexical Environment
- Creating Your First Closure
- Common Use Cases for Closures
- Closures in Event Handlers
- Advanced Closure Patterns
- Real-World Applications
- Common Closure Pitfalls
- Debugging Closures
- Closures in Modern JavaScript
- Best Practices
- Conclusion
Introduction
JavaScript closures often appear mysterious to developers, yet they’re one of the language’s most powerful features. Whether you’re building complex web applications, working with event handlers, or implementing design patterns, understanding closures is essential for writing clean, efficient JavaScript code.
In this comprehensive guide, we’ll demystify closures with clear explanations and practical examples. By the end, you’ll not only understand what closures are, but you’ll also know how to leverage them effectively in your real-world projects.
What is a Closure in JavaScript?
A closure is a function that remembers and accesses variables from its outer scope, even after the outer function has finished executing. In simpler terms, closures give you access to an outer function’s scope from an inner function.
This concept might sound abstract, but it’s fundamental to how JavaScript works and leads to powerful programming patterns.
How Closures Work: Scope and Lexical Environment
To understand closures, you need to grasp two key concepts:
- Lexical Scoping: Variables are resolved based on their location in the source code.
- Execution Context: Each function creates its own execution context with a variable environment.
When a function is created, it forms a closure over the current variables in scope. This means the function “closes over” these variables, maintaining access to them even when executed elsewhere.
function outerFunction() {
const message = 'Hello, closures!';
function innerFunction() {
console.log(message); // Access variable from outer scope
}
return innerFunction;
}
const myFunction = outerFunction();
myFunction(); // Output: "Hello, closures!"
In this example, innerFunction
maintains access to the message
variable even after outerFunction
has completed execution. This is a closure in action.
Creating Your First Closure
Let’s build a simple counter using closures:
function createCounter() {
let count = 0;
return {
increment: function() {
count++;
return count;
},
decrement: function() {
count--;
return count;
},
getValue: function() {
return count;
}
};
}
const counter = createCounter();
console.log(counter.increment()); // 1
console.log(counter.increment()); // 2
console.log(counter.decrement()); // 1
console.log(counter.getValue()); // 1
The beauty of this pattern is that the count
variable is completely private. It can’t be accessed or modified directly from outside the createCounter
function. The only way to interact with it is through the methods provided.
Common Use Cases for Closures
Data Privacy and Encapsulation
Closures provide a way to create private variables in JavaScript, a language that doesn’t have built-in privacy modifiers like some other languages:
function createBankAccount(initialBalance) {
let balance = initialBalance; // Private variable
return {
deposit: function(amount) {
if (amount > 0) {
balance += amount;
return `Deposited ${amount}. New balance: ${balance}`;
}
return 'Invalid deposit amount';
},
withdraw: function(amount) {
if (amount > 0 && amount <= balance) {
balance -= amount;
return `Withdrew ${amount}. New balance: ${balance}`;
}
return 'Invalid withdrawal amount or insufficient funds';
},
getBalance: function() {
return `Current balance: ${balance}`;
}
};
}
const account = createBankAccount(100);
console.log(account.getBalance()); // "Current balance: 100"
console.log(account.deposit(50)); // "Deposited 50. New balance: 150"
console.log(account.withdraw(30)); // "Withdrew 30. New balance: 120"
console.log(account.balance); // undefined - can't access private variable
In this example, the balance
variable is protected from external access, enforcing data integrity through the provided methods.
Function Factories
Closures enable the creation of specialized functions based on parameters:
function createMultiplier(multiplier) {
return function(number) {
return number * multiplier;
};
}
const double = createMultiplier(2);
const triple = createMultiplier(3);
console.log(double(5)); // 10
console.log(triple(5)); // 15
This pattern is powerful when you need functions with similar behavior but different configurations.
Maintaining State in Callbacks
Closures are essential when working with callbacks, allowing you to preserve state between function calls:
function fetchDataWithRetry(url, maxRetries = 3) {
let retryCount = 0;
function attemptFetch() {
fetch(url)
.then(response => {
console.log('Data fetched successfully');
// Process response
})
.catch(error => {
retryCount++; // The closure remembers this count
console.log(`Attempt ${retryCount} failed`);
if (retryCount < maxRetries) {
console.log(`Retrying... (${maxRetries - retryCount} attempts left)`);
setTimeout(attemptFetch, 1000 * retryCount); // Exponential backoff
} else {
console.error('Max retries reached. Giving up.');
}
});
}
attemptFetch();
}
fetchDataWithRetry('https://api.example.com/data');
The retryCount
variable persists across asynchronous function calls, maintaining the state of how many retries have been attempted.
Implementing Module Pattern
Before ES6 modules, the module pattern was the standard way to create reusable, encapsulated code in JavaScript:
const shoppingCartModule = (function() {
// Private variables
let items = [];
let total = 0;
// Private method
function calculateTotal() {
return items.reduce((sum, item) => sum + item.price * item.quantity, 0);
}
// Public API
return {
addItem: function(name, price, quantity = 1) {
items.push({ name, price, quantity });
total = calculateTotal();
return `${name} added to cart`;
},
removeItem: function(name) {
const index = items.findIndex(item => item.name === name);
if (index !== -1) {
items.splice(index, 1);
total = calculateTotal();
return `${name} removed from cart`;
}
return `${name} not found in cart`;
},
getCartSummary: function() {
return {
items: [...items], // Return copy to prevent mutation
itemCount: items.reduce((sum, item) => sum + item.quantity, 0),
total: total
};
}
};
})();
console.log(shoppingCartModule.addItem('Laptop', 999.99)); // "Laptop added to cart"
console.log(shoppingCartModule.addItem('Mouse', 29.99, 2)); // "Mouse added to cart"
console.log(shoppingCartModule.getCartSummary());
// {items: [{name: "Laptop", price: 999.99, quantity: 1}, {name: "Mouse", price: 29.99, quantity: 2}], itemCount: 3, total: 1059.97}
This pattern creates a module with private variables and methods, exposing only a public API through closures.
Memoization for Performance
Closures can optimize performance by caching results of expensive function calls:
function createFibonacciCalculator() {
// Private cache
const cache = {};
function fibonacci(n) {
// Check if result is already cached
if (n in cache) {
console.log(`Using cached result for fibonacci(${n})`);
return cache[n];
}
// Calculate for the first time
console.log(`Calculating fibonacci(${n})`);
let result;
if (n <= 1) {
result = n;
} else {
result = fibonacci(n - 1) + fibonacci(n - 2);
}
// Store in cache for future use
cache[n] = result;
return result;
}
return fibonacci;
}
const fib = createFibonacciCalculator();
console.log(fib(6)); // Calculates and caches all values from 0-6
console.log(fib(7)); // Only needs to calculate fib(7), reuses cached values
Each time the function runs, it stores results in the cache
object, avoiding redundant calculations in future calls.
Closures in Event Handlers
Closures are essential when working with event handlers, allowing you to access data at the time the handler is created:
function setupButtonHandlers() {
const buttons = document.querySelectorAll('.product-button');
buttons.forEach(button => {
const productId = button.dataset.productId;
const productName = button.dataset.productName;
const productPrice = parseFloat(button.dataset.productPrice);
button.addEventListener('click', function() {
// Closure retains access to productId, productName, and productPrice
console.log(`Adding ${productName} to cart`);
addToCart({
id: productId,
name: productName,
price: productPrice
});
});
});
}
function addToCart(product) {
console.log(`Product added to cart:`, product);
// Implementation of adding to cart
}
// Call this when the page loads
setupButtonHandlers();
Each button’s click handler forms a closure over the specific product details, ensuring that the correct product is added to the cart even if the DOM changes later.
Advanced Closure Patterns
Partial Application and Currying
Closures enable functional programming techniques like partial application and currying:
// Currying with closures
function curry(fn) {
return function curried(...args) {
if (args.length >= fn.length) {
return fn.apply(this, args);
} else {
return function(...moreArgs) {
return curried.apply(this, args.concat(moreArgs));
};
}
};
}
// A function to be curried
function calculateTotal(basePrice, taxRate, discount) {
return (basePrice + (basePrice * taxRate)) - discount;
}
const curriedCalculation = curry(calculateTotal);
// Create specialized functions using partial application
const calculateWithStandardTax = curriedCalculation(100)(0.1);
console.log(calculateWithStandardTax(20)); // (100 + 10) - 20 = 90
// Another specialized function
const luxuryItemWithTax = curriedCalculation(500)(0.2);
console.log(luxuryItemWithTax(50)); // (500 + 100) - 50 = 550
This pattern allows you to create specialized functions by fixing certain parameters, improving code reusability.
Implementing Private Methods
Closures can be used to create private methods in JavaScript classes:
class User {
constructor(name, email) {
this.name = name;
this.email = email;
// Private methods using closures
const validateEmail = (email) => {
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return emailRegex.test(email);
};
const generateUserId = () => {
return Math.random().toString(36).substr(2, 9);
};
// Private properties
const userId = generateUserId();
// Public methods that can access private methods and properties
this.isValidUser = () => {
return validateEmail(this.email) && this.name.length > 0;
};
this.getUserData = () => {
return {
id: userId, // Accessing private property
name: this.name,
email: this.email,
valid: validateEmail(this.email) // Using private method
};
};
}
}
const user = new User('John Doe', 'john@example.com');
console.log(user.isValidUser()); // true
console.log(user.getUserData());
// {id: "x7f8d9e2", name: "John Doe", email: "john@example.com", valid: true}
// Can't access private methods or properties
console.log(user.validateEmail); // undefined
console.log(user.userId); // undefined
Higher-Order Functions
Closures are at the core of higher-order functions, which are functions that take or return other functions:
// A debounce function that uses closures to limit function calls
function debounce(func, delay) {
let timeoutId;
return function(...args) {
const context = this;
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
func.apply(context, args);
}, delay);
};
}
// Usage example
const handleSearch = debounce(function(query) {
console.log(`Searching for: ${query}`);
// API call or search logic here
}, 500);
// In a real UI, this would be called on input events
handleSearch('JavaScript');
handleSearch('JavaScript c'); // Called 100ms later
handleSearch('JavaScript closures'); // Called 200ms later
// Only the last call will actually execute after the 500ms delay
The debounce
function creates a closure that maintains the state of the timeoutId
, allowing it to track and clear previous timeouts when new calls are made.
Real-World Applications
Building a Shopping Cart
Let’s build a more complete shopping cart implementation using closures:
function createShoppingCart() {
// Private state
const items = [];
let totalPrice = 0;
// Private methods
function findItemIndex(productId) {
return items.findIndex(item => item.product.id === productId);
}
function recalculateTotal() {
totalPrice = items.reduce((sum, item) =>
sum + (item.product.price * item.quantity), 0);
}
function formatCurrency(amount) {
return `$${amount.toFixed(2)}`;
}
// Public API
return {
addItem(product, quantity = 1) {
const existingIndex = findItemIndex(product.id);
if (existingIndex >= 0) {
// Update existing item
items[existingIndex].quantity += quantity;
} else {
// Add new item
items.push({
product: { ...product }, // Clone to prevent external mutation
quantity
});
}
recalculateTotal();
return {
message: `Added ${quantity} x ${product.name} to cart`,
itemCount: this.getItemCount()
};
},
removeItem(productId, quantity = null) {
const existingIndex = findItemIndex(productId);
if (existingIndex === -1) {
return {
message: `Product not found in cart`,
itemCount: this.getItemCount()
};
}
const item = items[existingIndex];
// If quantity is null or >= item quantity, remove entire item
if (quantity === null || quantity >= item.quantity) {
items.splice(existingIndex, 1);
recalculateTotal();
return {
message: `Removed ${item.product.name} from cart`,
itemCount: this.getItemCount()
};
} else {
// Reduce quantity
item.quantity -= quantity;
recalculateTotal();
return {
message: `Reduced ${item.product.name} quantity by ${quantity}`,
itemCount: this.getItemCount()
};
}
},
getCartContents() {
// Return deep clone to prevent mutations
return JSON.parse(JSON.stringify({
items,
totalPrice,
formattedTotal: formatCurrency(totalPrice)
}));
},
getItemCount() {
return items.reduce((count, item) => count + item.quantity, 0);
},
clearCart() {
items.length = 0;
totalPrice = 0;
return {
message: 'Cart cleared',
itemCount: 0
};
}
};
}
// Usage
const cart = createShoppingCart();
console.log(cart.addItem({ id: 'p1', name: 'JavaScript Book', price: 39.99 }));
console.log(cart.addItem({ id: 'p2', name: 'Mechanical Keyboard', price: 89.99 }, 1));
console.log(cart.addItem({ id: 'p1', name: 'JavaScript Book', price: 39.99 }, 1)); // Add another of p1
console.log(cart.getCartContents());
// {
// items: [
// {product: {id: "p1", name: "JavaScript Book", price: 39.99}, quantity: 2},
// {product: {id: "p2", name: "Mechanical Keyboard", price: 89.99}, quantity: 1}
// ],
// totalPrice: 169.97,
// formattedTotal: "$169.97"
// }
console.log(cart.removeItem('p1', 1)); // Remove one book
console.log(cart.getCartContents());
This shopping cart implementation uses closures to maintain private state and methods, providing a clean public API while preventing external code from directly manipulating the cart’s contents.
Creating a Countdown Timer
Let’s implement a reusable countdown timer using closures:
function createCountdownTimer() {
let timerId = null;
let endTime = null;
let callbacks = {
tick: null,
complete: null
};
// Private method
function calculateTimeRemaining() {
const now = new Date().getTime();
const distance = endTime - now;
if (distance < 0) {
return {
days: 0,
hours: 0,
minutes: 0,
seconds: 0,
completed: true
};
}
// Time calculations
const days = Math.floor(distance / (1000 * 60 * 60 * 24));
const hours = Math.floor((distance % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((distance % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((distance % (1000 * 60)) / 1000);
return {
days,
hours,
minutes,
seconds,
completed: false
};
}
// Private method
function updateTimer() {
const timeRemaining = calculateTimeRemaining();
if (callbacks.tick) {
callbacks.tick(timeRemaining);
}
if (timeRemaining.completed) {
clearInterval(timerId);
timerId = null;
if (callbacks.complete) {
callbacks.complete();
}
}
}
// Public API
return {
start(durationInSeconds) {
// Stop any existing timer
this.stop();
// Set end time
endTime = new Date().getTime() + (durationInSeconds * 1000);
// Update immediately
updateTimer();
// Set interval
timerId = setInterval(updateTimer, 1000);
return this;
},
stop() {
if (timerId !== null) {
clearInterval(timerId);
timerId = null;
}
return this;
},
onTick(callback) {
callbacks.tick = callback;
return this;
},
onComplete(callback) {
callbacks.complete = callback;
return this;
},
getTimeRemaining() {
return calculateTimeRemaining();
},
isRunning() {
return timerId !== null;
}
};
}
// Usage example
const timer = createCountdownTimer();
timer
.onTick(time => {
console.log(`${time.minutes}:${time.seconds.toString().padStart(2, '0')}`);
})
.onComplete(() => {
console.log('Countdown finished!');
// Could trigger other actions here
})
.start(5); // 5 second countdown
In this example, the timer uses closures to maintain its state (timerId, endTime, callbacks) privately while exposing a clean, chainable API.
Implementing a Custom React-like Hook
Let’s implement a simple state management hook inspired by React’s useState, using closures:
function createStateHook(initialValue) {
// State is enclosed in the closure
let state = initialValue;
const listeners = [];
function setState(newValue) {
// Option to use a function to update state
if (typeof newValue === 'function') {
state = newValue(state);
} else {
state = newValue;
}
// Notify all listeners
listeners.forEach(listener => listener(state));
}
function getState() {
return state;
}
function subscribe(listener) {
listeners.push(listener);
// Return unsubscribe function
return function unsubscribe() {
const index = listeners.indexOf(listener);
if (index !== -1) {
listeners.splice(index, 1);
}
};
}
// Return the hook API
return [getState, setState, subscribe];
}
// Example usage
const [getCount, setCount, subscribeToCount] = createStateHook(0);
// Subscribe to changes
const unsubscribe = subscribeToCount(count => {
console.log(`Count changed to: ${count}`);
});
// Update the state
setCount(1); // "Count changed to: 1"
setCount(count => count + 1); // "Count changed to: 2"
// Get current state
console.log(getCount()); // 2
// Unsubscribe when done
unsubscribe();
// No more logs after unsubscribing
setCount(3);
This demonstrates how closures can be used to build state management systems similar to those in modern frameworks.
Common Closure Pitfalls
Memory Management
Closures can lead to memory leaks if not used carefully:
function setupEventWithLeak() {
const largeData = new Array(1000000).fill('potentially large data');
document.getElementById('myButton').addEventListener('click', function() {
// This closure captures the entire largeData array
console.log('Button clicked!', largeData.length);
});
}
// Better approach
function setupEventWithoutLeak() {
// Set up event listener
document.getElementById('myButton').addEventListener('click', handleClick);
function handleClick() {
// Only access what you need, don't capture large data
console.log('Button clicked, no leak!');
}
// Process large data here, but don't capture it in the closure
const largeData = new Array(1000000).fill('potentially large data');
processData(largeData);
}
Always be mindful of what variables your closures are capturing, especially with event listeners that may persist for the lifetime of the page.
Loop Variables in Closures
A classic closure pitfall occurs with loop variables:
// Problematic code
function createButtons() {
for (var i = 0; i < 5; i++) {
const button = document.createElement('button');
button.textContent = `Button ${i}`;
button.addEventListener('click', function() {
console.log(`Button ${i} clicked`); // Will always log "Button 5 clicked"
});
document.body.appendChild(button);
}
}
// Fixed version using let (block scoped)
function createButtonsFixed() {
for (let i = 0; i < 5; i++) {
const button = document.createElement('button');
button.textContent = `Button ${i}`;
button.addEventListener('click', function() {
console.log(`Button ${i} clicked`); // Correctly logs the button number
});
document.body.appendChild(button);
}
}
// Alternative fix using an IIFE to create a new scope
function createButtonsWithIIFE() {
for (var i = 0; i < 5; i++) {
(function(buttonIndex) {
const button = document.createElement('button');
button.textContent = `Button ${buttonIndex}`;
button.addEventListener('click', function() {
console.log(`Button ${buttonIndex} clicked`); // Correctly logs button number
});
document.body.appendChild(button);
})(i);
}
}
This issue was common in pre-ES6 JavaScript. Using let
in loops creates a new binding for each iteration, solving the problem elegantly.
Performance Considerations
While closures are powerful, they do come with some performance overhead:
// Less optimal - creates a new function for each item
function processList(items) {
return items.map(item => {
return processItem(item); // New closure for each item
});
}
// More optimal - reuses the same function
function processList(items) {
return items.map(processItem); // Reuse the same function
}
function processItem(item) {
return item.value * 2;
}
When working with large data sets or performance-critical code, be mindful of unnecessarily creating closures.
Debugging Closures
Debugging closures can be challenging. Here are some techniques to help:
function createCounter(label) {
let count = 0;
// Add debugging information
console.log(`Creating counter: ${label}`);
return function() {
count++;
console.log(`${label} counter: ${count}`);
debugger; // Pause execution to inspect in browser devtools
return count;
};
}
const counterA = createCounter('A');
const counterB = createCounter('B');
counterA(); // "A counter: 1"
counterA(); // "A counter: 2"
counterB(); // "B counter: 1"
Modern browser developer tools provide scope inspection that can help visualize closure variables during debugging.
Closures in Modern JavaScript
Modern JavaScript has evolved with features that complement closures:
// Using closures with arrow functions
const createAdder = base => num => base + num;
const addFive = createAdder(5);
console.log(addFive(10)); // 15
// Using closures with destructuring and default parameters
const createFormatter = (
{ separator = ',', currency = '$', decimals = 2 } = {}
) => {
return num => {
return `${currency}${num.toFixed(decimals).replace('.', separator)}`;
};
};
const formatUSD = createFormatter();
const formatEUR = createFormatter({ currency: '€', separator: ',' });
console.log(formatUSD(1234.5)); // "$1234.50"
console.log(formatEUR(1234.5)); // "€1234,50"
// Using closures with ES modules
// file: counter.js
let count = 0; // Private through closure in module scope
export function increment() {
return ++count;
}
export function decrement() {
return --count;
}
export function getCount() {
return count;
}
// usage in another file
// import { increment, getCount } from './counter.js';
// console.log(increment()); // 1
// console.log(getCount()); // 1
ES modules provide a clean way to achieve encapsulation similar to closures at the module level.
Best Practices
Here are some closure best practices:
- Keep it simple: Only capture the variables you need in a closure
- Avoid excessive nesting: Flatten code when possible for better readability
- Be mindful of memory: Release references when no longer needed
- Document closure dependencies: Make it clear what external variables a function depends on
- Use ES6 features: Leverage arrow functions, let/const, and modules with closures
- Use clear naming conventions: Especially for factory functions that create closures
Conclusion
JavaScript closures are a powerful mechanism that enables advanced patterns like data privacy, function factories, and stateful functions. By maintaining access to their lexical environment, closures allow for elegant solutions to complex programming challenges.
As you’ve seen in this guide, closures are not just a theoretical concept but a practical tool that you’ll use daily when writing modern JavaScript applications. From simple counters to complex state management systems, closures are the secret ingredient that makes JavaScript so flexible and powerful.
Now that you understand how closures work and have seen real-world applications, you’re equipped to leverage this powerful JavaScript feature in your own projects. Experiment with the patterns we’ve covered, and you’ll discover even more ways to use closures to write cleaner, more maintainable code.
Comments