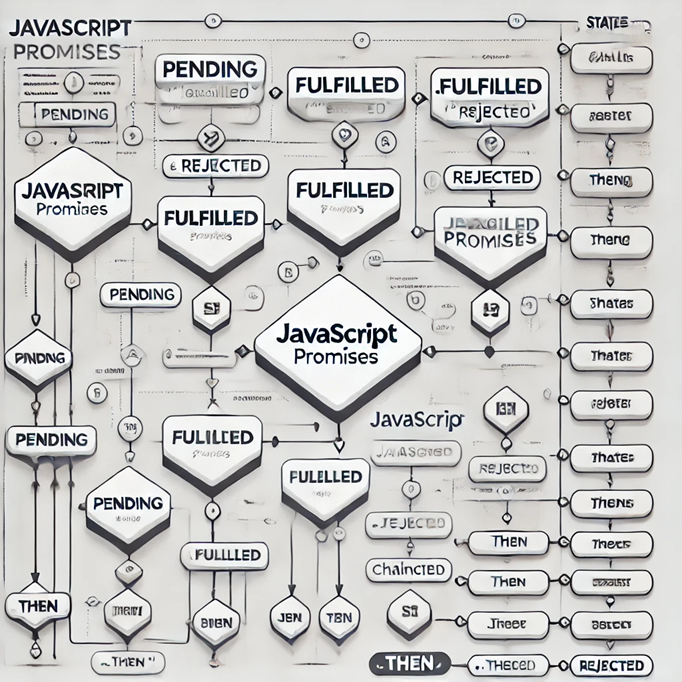
JavaScript Promises: The Complete Guide to Asynchronous Programming [2025]
JavaScript Promises: A Complete Guide to Asynchronous Programming
Table of Contents
- Introduction
- What are JavaScript Promises?
- Why Use Promises?
- Creating Your First Promise
- Promise States and Fates
- Promise Chaining with .then()
- Error Handling with .catch()
- The .finally() Method
- Promise.all() for Parallel Execution
- Promise.race()
- Promise.allSettled()
- Promise.any()
- Async/Await: A Cleaner Approach
- Real-World Use Cases
- Common Promise Mistakes to Avoid
- Debugging Promises
- Browser Compatibility
- Conclusion
Introduction
Have you ever been frustrated by JavaScript code that gets messy with multiple nested callbacks? Or struggled with handling asynchronous operations elegantly? JavaScript Promises are the solution you’ve been looking for.
In today’s web development landscape, asynchronous programming is unavoidable. From fetching API data to handling user interactions, understanding how to manage asynchronous code efficiently can make the difference between smooth, responsive applications and slow, buggy ones.
This comprehensive guide will take you from Promise basics to advanced techniques, complete with practical examples you can start using in your projects today.
What are JavaScript Promises?
A Promise in JavaScript is an object representing the eventual completion or failure of an asynchronous operation. Think of it as a “promise” to return a value at some point in the future.
Before promises were introduced in ES6 (2015), developers relied on callbacks, which often led to deeply nested code known as “callback hell” or the “pyramid of doom.”
// The old way (callback hell)
fetchData(function(data) {
processData(data, function(processedData) {
saveData(processedData, function(result) {
displayData(result, function() {
console.log("Finally done!");
});
});
});
});
Promises help flatten this structure and make asynchronous code more readable and maintainable.
Why Use Promises?
Promises offer several key advantages:
- Improved readability: Chain operations instead of nesting them
- Better error handling: Centralized error handling with
.catch()
- Flow control: Execute asynchronous operations in sequence or parallel
- Composability: Combine promises for complex asynchronous workflows
- Predictability: Promises are always asynchronous, avoiding timing issues
Creating Your First Promise
Creating a promise is straightforward. The Promise constructor takes a single argument: a function (called the “executor”) with two parameters - resolve
and reject
:
const myPromise = new Promise((resolve, reject) => {
// Asynchronous operation here
const success = true; // This would be your condition
if (success) {
resolve("Operation completed successfully!");
} else {
reject("Operation failed!");
}
});
Let’s create a practical example. Imagine we’re simulating a database query with a slight delay:
function fetchUserData(userId) {
return new Promise((resolve, reject) => {
// Simulate network request
setTimeout(() => {
// Fake database
const users = {
1: { id: 1, name: "John Doe", email: "john@example.com" },
2: { id: 2, name: "Jane Smith", email: "jane@example.com" }
};
const user = users[userId];
if (user) {
resolve(user);
} else {
reject(`User with ID ${userId} not found.`);
}
}, 1000);
});
}
// Usage
fetchUserData(1)
.then(user => console.log("User found:", user))
.catch(error => console.error("Error:", error));
Promise States and Fates
Promises have three possible states:
- Pending: Initial state, neither fulfilled nor rejected
- Fulfilled: Operation completed successfully
- Rejected: Operation failed
Once a promise is fulfilled or rejected, it becomes “settled” and cannot change to another state. This immutability is key to understanding Promises.
Promise Chaining with .then()
One of the most powerful features of promises is the ability to chain operations. Each .then()
returns a new promise, allowing you to create a sequence of asynchronous operations:
fetchUserData(1)
.then(user => {
console.log("User:", user);
return fetchUserPosts(user.id); // Returns another promise
})
.then(posts => {
console.log("User's posts:", posts);
return fetchPostComments(posts[0].id); // Returns another promise
})
.then(comments => {
console.log("Comments on first post:", comments);
})
.catch(error => {
console.error("Error in the chain:", error);
});
Let’s implement a complete example with this chaining pattern:
// Simulate fetching user data
function fetchUserData(userId) {
return new Promise((resolve, reject) => {
setTimeout(() => {
const users = {
1: { id: 1, name: "John Doe", email: "john@example.com" }
};
const user = users[userId];
if (user) {
resolve(user);
} else {
reject(`User with ID ${userId} not found.`);
}
}, 1000);
});
}
// Simulate fetching user posts
function fetchUserPosts(userId) {
return new Promise((resolve, reject) => {
setTimeout(() => {
const posts = {
1: [
{ id: 101, title: "Understanding JavaScript Promises" },
{ id: 102, title: "Advanced CSS Techniques" }
]
};
const userPosts = posts[userId];
if (userPosts) {
resolve(userPosts);
} else {
reject(`Posts for user ${userId} not found.`);
}
}, 1000);
});
}
// Simulate fetching post comments
function fetchPostComments(postId) {
return new Promise((resolve, reject) => {
setTimeout(() => {
const comments = {
101: [
{ id: 1001, text: "Great article!", user: "Alice" },
{ id: 1002, text: "Very helpful, thanks!", user: "Bob" }
]
};
const postComments = comments[postId];
if (postComments) {
resolve(postComments);
} else {
reject(`Comments for post ${postId} not found.`);
}
}, 1000);
});
}
// Chain all operations
fetchUserData(1)
.then(user => {
console.log("Found user:", user.name);
return fetchUserPosts(user.id);
})
.then(posts => {
console.log("User has", posts.length, "posts");
return fetchPostComments(posts[0].id);
})
.then(comments => {
console.log("First post has", comments.length, "comments");
console.log("Comments:", comments);
})
.catch(error => {
console.error("Error in the chain:", error);
});
In this example, we’re:
- Fetching a user
- Using that user’s ID to fetch their posts
- Using the first post’s ID to fetch comments
- Handling any errors in the entire process with a single
.catch()
Error Handling with .catch()
Proper error handling is crucial for robust applications. The .catch()
method provides a convenient way to handle errors in promise chains:
fetchData()
.then(processData)
.then(saveData)
.then(displayData)
.catch(error => {
console.error("An error occurred:", error);
showErrorToUser(error); // Show user-friendly error message
});
You can also place multiple .catch()
handlers at different points in your chain to handle specific errors:
fetchUserData(999) // Using non-existent ID
.then(user => {
console.log("User:", user);
return fetchUserPosts(user.id);
})
.catch(error => {
console.error("Error fetching user:", error);
return { id: 0, name: "Guest" }; // Provide default user and continue
})
.then(user => {
return fetchUserPosts(user.id);
})
.then(posts => {
console.log("Posts:", posts);
})
.catch(error => {
console.error("Error fetching posts:", error);
});
The .finally() Method
The .finally()
method allows you to execute code regardless of whether the promise was fulfilled or rejected. It’s perfect for cleanup operations:
showLoadingIndicator();
fetchData()
.then(data => {
console.log("Data received:", data);
})
.catch(error => {
console.error("Error:", error);
})
.finally(() => {
hideLoadingIndicator(); // This runs regardless of success or failure
});
Promise.all() for Parallel Execution
When you need to run multiple asynchronous operations in parallel and wait for all of them to complete, Promise.all()
is your friend:
// Fetch multiple resources in parallel
Promise.all([
fetchUserProfile(userId),
fetchUserPosts(userId),
fetchUserFollowers(userId)
])
.then(([profile, posts, followers]) => {
// All three promises have resolved
console.log("Profile:", profile);
console.log("Posts:", posts);
console.log("Followers:", followers);
// Now update the UI with all data
updateProfileUI(profile, posts, followers);
})
.catch(error => {
// If ANY promise rejects, this will execute
console.error("Failed to fetch all user data:", error);
});
Real-world example with the Fetch API:
// Fetch data from multiple endpoints simultaneously
Promise.all([
fetch('https://api.example.com/users').then(response => response.json()),
fetch('https://api.example.com/posts').then(response => response.json()),
fetch('https://api.example.com/comments').then(response => response.json())
])
.then(([users, posts, comments]) => {
// Process all the data
const enhancedPosts = posts.map(post => {
return {
...post,
author: users.find(user => user.id === post.userId),
comments: comments.filter(comment => comment.postId === post.id)
};
});
console.log("Enhanced posts:", enhancedPosts);
renderPosts(enhancedPosts);
})
.catch(error => {
console.error("Error fetching data:", error);
showErrorMessage("Failed to load content. Please try again later.");
});
Promise.race()
While Promise.all()
waits for all promises to resolve, Promise.race()
resolves or rejects as soon as the first promise in the array settles:
// Implement a timeout for an API call
function fetchWithTimeout(url, timeout = 5000) {
return Promise.race([
fetch(url).then(response => response.json()),
new Promise((_, reject) =>
setTimeout(() => reject(new Error("Request timed out")), timeout)
)
]);
}
// Usage
fetchWithTimeout('https://api.example.com/data', 3000)
.then(data => console.log("Data received:", data))
.catch(error => console.error("Error:", error.message));
This pattern is useful for implementing timeouts or taking the result of whichever operation completes first.
Promise.allSettled()
Introduced in ES2020, Promise.allSettled()
waits for all promises to settle, regardless of whether they fulfill or reject:
Promise.allSettled([
fetch('https://api.example.com/endpoint1').then(r => r.json()),
fetch('https://api.example.com/endpoint2').then(r => r.json()),
fetch('https://api.example.com/endpoint3').then(r => r.json())
])
.then(results => {
// Check the status of each promise
results.forEach((result, index) => {
if (result.status === 'fulfilled') {
console.log(`Request ${index + 1} succeeded:`, result.value);
} else {
console.log(`Request ${index + 1} failed:`, result.reason);
}
});
// Process the successful results
const successfulData = results
.filter(result => result.status === 'fulfilled')
.map(result => result.value);
console.log("All successful data:", successfulData);
});
This is particularly useful when you want to attempt multiple operations and continue with whatever succeeded, rather than failing if any single operation fails.
Promise.any()
Promise.any()
(ES2021) resolves as soon as any of the promises in the array fulfills, or rejects if all promises reject:
// Try to fetch data from multiple fallback APIs
Promise.any([
fetch('https://primary-api.example.com/data').then(r => r.json()),
fetch('https://backup1-api.example.com/data').then(r => r.json()),
fetch('https://backup2-api.example.com/data').then(r => r.json())
])
.then(data => {
console.log("Data received from first available API:", data);
updateUI(data);
})
.catch(errors => {
// AggregateError contains all the individual errors
console.error("All APIs failed:", errors);
showErrorMessage("Cannot retrieve data at this time");
});
This is perfect for implementing fallback logic when you have multiple ways to accomplish the same task.
Async/Await: A Cleaner Approach
While promises are powerful, the async/await syntax introduced in ES2017 makes asynchronous code even cleaner and more intuitive:
async function getUserDataComplete(userId) {
try {
// Each await pauses execution until the promise resolves
const user = await fetchUserData(userId);
console.log("User:", user);
const posts = await fetchUserPosts(user.id);
console.log("Posts:", posts);
const comments = await fetchPostComments(posts[0].id);
console.log("Comments:", comments);
return {
user,
posts,
comments
};
} catch (error) {
console.error("Error:", error);
throw error; // Re-throw or handle appropriately
}
}
// Usage (remember this returns a promise!)
getUserDataComplete(1)
.then(result => {
console.log("Everything loaded successfully:", result);
displayUserProfile(result);
})
.catch(error => {
showErrorMessage("Failed to load user profile");
});
You can also use async/await with Promise.all for parallel execution:
async function loadDashboardData(userId) {
try {
// Run these requests in parallel
const [profile, posts, analytics] = await Promise.all([
fetchUserProfile(userId),
fetchUserPosts(userId),
fetchUserAnalytics(userId)
]);
return {
profile,
posts,
analytics
};
} catch (error) {
console.error("Failed to load dashboard:", error);
throw error;
}
}
Real-World Use Cases
Let’s explore some practical applications of promises:
1. Image Preloader
function preloadImage(url) {
return new Promise((resolve, reject) => {
const img = new Image();
img.src = url;
img.onload = () => resolve(img);
img.onerror = () => reject(new Error(`Failed to load image: ${url}`));
});
}
// Preload multiple images
Promise.all([
preloadImage('image1.jpg'),
preloadImage('image2.jpg'),
preloadImage('image3.jpg')
])
.then(images => {
console.log("All images preloaded!");
// Add images to the DOM
images.forEach(img => document.body.appendChild(img));
})
.catch(error => {
console.error("Image preloading failed:", error);
});
2. Sequential API Requests with Retry Logic
async function fetchWithRetry(url, options = {}, retries = 3, delay = 1000) {
try {
const response = await fetch(url, options);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return await response.json();
} catch (error) {
if (retries > 0) {
console.log(`Retrying... Attempts left: ${retries}`);
// Wait before retrying
await new Promise(resolve => setTimeout(resolve, delay));
// Try again with one fewer retry
return fetchWithRetry(url, options, retries - 1, delay * 1.5);
} else {
// Out of retries, rethrow the error
throw error;
}
}
}
// Usage
fetchWithRetry('https://api.example.com/data')
.then(data => console.log("Data:", data))
.catch(error => console.error("Final error:", error));
3. Rate-Limited API Requests
async function fetchWithRateLimit(urls, maxConcurrent = 3, delayMs = 100) {
const results = [];
// Process URLs in chunks to respect rate limits
for (let i = 0; i < urls.length; i += maxConcurrent) {
const chunk = urls.slice(i, i + maxConcurrent);
// Process this chunk in parallel
const chunkResults = await Promise.all(
chunk.map(url => fetch(url).then(r => r.json()))
);
// Add results from this chunk
results.push(...chunkResults);
// Wait before processing the next chunk
if (i + maxConcurrent < urls.length) {
await new Promise(resolve => setTimeout(resolve, delayMs));
}
}
return results;
}
// Example usage
const apiUrls = [
'https://api.example.com/items/1',
'https://api.example.com/items/2',
// ...more URLs
];
fetchWithRateLimit(apiUrls)
.then(results => console.log("All data:", results))
.catch(error => console.error("Error:", error));
Common Promise Mistakes to Avoid
1. Forgetting to Return Promises in Chains
// INCORRECT
function processUserData(userId) {
fetchUserData(userId)
.then(user => {
// This promise result is lost!
fetchUserPosts(user.id);
})
.then(posts => {
// 'posts' will be undefined!
console.log(posts);
});
}
// CORRECT
function processUserData(userId) {
return fetchUserData(userId)
.then(user => {
// Return the promise to continue the chain
return fetchUserPosts(user.id);
})
.then(posts => {
// Now 'posts' will contain the expected data
console.log(posts);
});
}
2. Nesting Promises Instead of Chaining
// INCORRECT - Creates "Promise Hell"
fetchUserData(userId).then(user => {
fetchUserPosts(user.id).then(posts => {
fetchPostComments(posts[0].id).then(comments => {
// We're back to callback hell!
console.log(comments);
});
});
});
// CORRECT - Flat Promise Chain
fetchUserData(userId)
.then(user => fetchUserPosts(user.id))
.then(posts => fetchPostComments(posts[0].id))
.then(comments => console.log(comments))
.catch(error => console.error(error));
3. Not Handling Errors Properly
// INCORRECT - Errors will be silently ignored
fetchData()
.then(processData)
.then(saveData);
// CORRECT - Always add error handling
fetchData()
.then(processData)
.then(saveData)
.catch(error => {
console.error("An error occurred:", error);
// Handle the error appropriately
});
Debugging Promises
Debugging asynchronous code can be challenging. Here are some tips:
1. Use Chrome DevTools Async Stack Traces
Modern browsers maintain the async call stack, making it easier to debug promises. In Chrome DevTools, you can see the full chain of async calls.
2. Add Descriptive Error Messages
function fetchUserData(userId) {
return fetch(`/api/users/${userId}`)
.then(response => {
if (!response.ok) {
throw new Error(`Failed to fetch user (ID: ${userId}). Status: ${response.status}`);
}
return response.json();
});
}
3. Log Intermediate States
fetchUserData(userId)
.then(user => {
console.log("User data received:", user);
return fetchUserPosts(user.id);
})
.then(posts => {
console.log("User posts received:", posts);
return fetchPostComments(posts[0].id);
})
.catch(error => {
console.error("Error in promise chain:", error);
});
Browser Compatibility
Modern promises are supported in all mainstream browsers, but if you need to support older browsers, consider using a polyfill like es6-promise.
For the newer promise methods:
Promise.all()
andPromise.race()
: Widely supported (IE11+ with polyfill)Promise.allSettled()
: Newer (may require polyfill)Promise.any()
: Most recent (requires polyfill for older browsers)
Conclusion
JavaScript Promises transform the way we write asynchronous code, making it more readable, maintainable, and robust. From basic chaining to advanced patterns like parallel execution and rate limiting, promises provide powerful tools for managing complex asynchronous workflows.
As you build more complex applications, mastering promises becomes essential. Combined with async/await syntax, you now have everything you need to write clean, efficient asynchronous JavaScript code.
Ready to level up your JavaScript skills further? Explore our other guides on InfoWorld360 for more in-depth JavaScript tutorials, including advanced topics like generators, observables, and reactive programming patterns.
Comments